Seeing Example
Describing an Image from a File
Suppose we want to get a description for this image
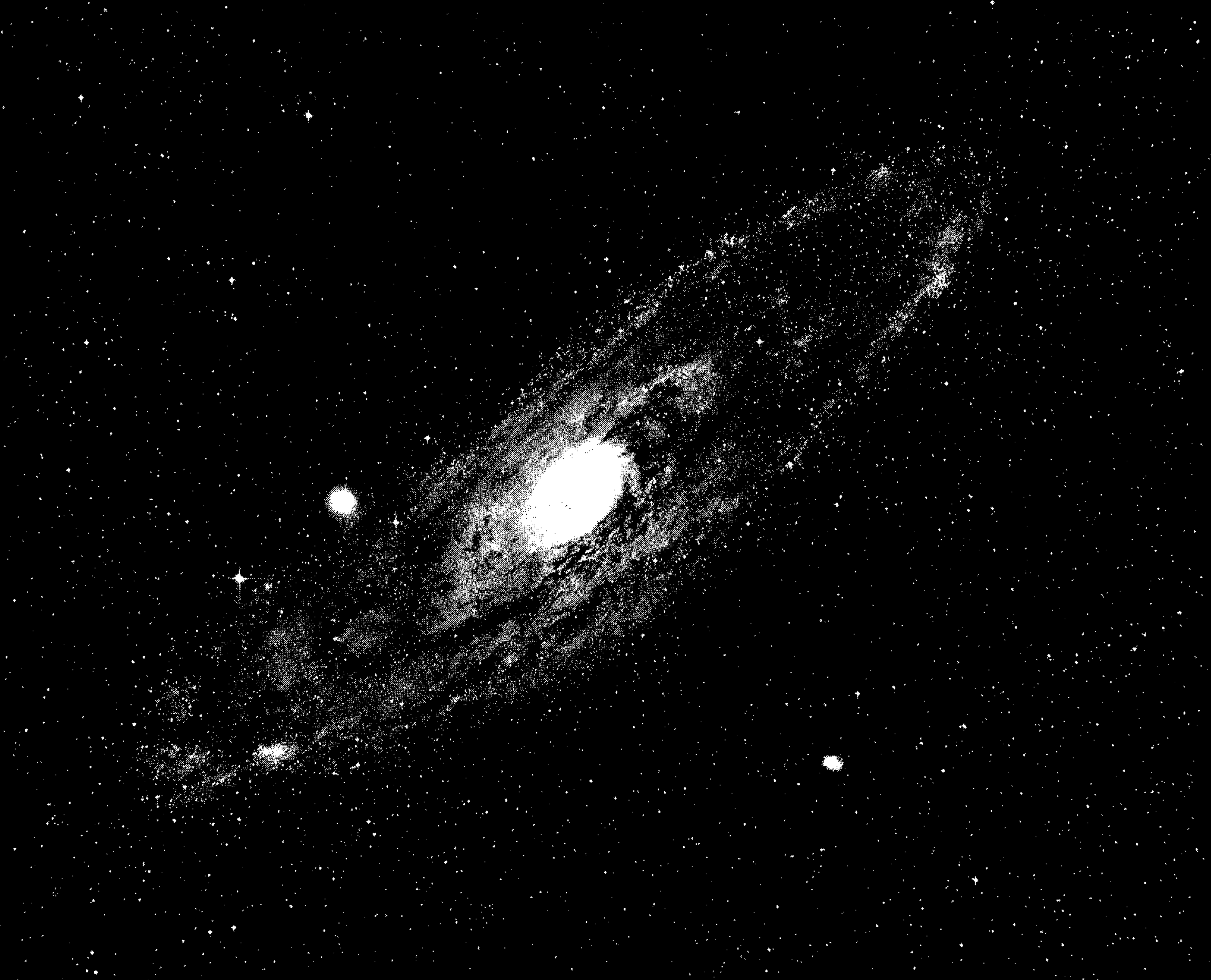
Python
import os
import base64
import requests
GEPPETTO_API_KEY = os.environ.get("GEPPETTO_API_KEY")
filename = "andromeda.png"
# Read file and construct dataURI
with open(filename, "rb") as file:
file_data = file.read()
data_uri = "data:image/png;base64," + base64.b64encode(file_data).decode("utf-8")
url = "https://api.geppetto.app/see"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {GEPPETTO_API_KEY}",
}
data = {
"image": data_uri
}
response = requests.post(url, headers=headers, json=data)
if response.status_code == 200:
print(response.json()["content"])
else:
print(f"Error: {response.status_code} - {response.text}")
This outputs the following description
The image depicts a spiral galaxy with a bright white center, surrounded by a vast
expanse of black space. The galaxy is positioned in the center of the frame.
The image is rendered in a black and white style, with the galaxy appearing as a
white dot against the black background. The image is slightly tilted to the
right, adding a dynamic element to the composition.
Node.js
import { Geppetto } from "@geppetto-app/geppetto";
import fs from "fs";
const geppetto = new Geppetto();
const filename = "andromeda.png";
async function main() {
// read file and construct dataURI
const file = fs.readFileSync(filename);
const dataURI = "data:image/png;base64," + file.toString("base64");
const response = await geppetto.see({
image: dataURI,
});
console.log(response.content);
}
main();
This outputs the same description as the Python example above.